Cron tasks
Utility to perform tasks based on a regular interval or any custom timing logic.
Usable even in scalable (multi-instance) applications thanks to task registration in MongoDB.
Mongodash will ensure each task is processed by only one instance at a time.
The task registration creates `cronTasks
collection in the database where all tasks are registered. You can see a short history of recent task runs here or even manually trigger a task if you need to!
cronTask(taskId, interval, taskFunction) => Promise
cronTask(taskId, interval, taskFunction) => Promise
Register a cron task.
const { cronTask } = require('mongodash');
cronTask('my-task-id', '5m 20s', async () => {
console.log('Hurray the task is running!');
});
import { cronTask } from 'mongodash';
cronTask('my-task-id', '5m 20s', async () => {
console.log('Hurray the task is running!');
});
That's it! Now the task will run every 5 minutes and 20 seconds!
First task registration also starts the internal loop watching for a task to run.
This can be prevented by calling stopCronTasks() or passing runCronTasks: false option to mongodash init.
Valid values for interval argument
Interval argument | Note | Example |
---|---|---|
interval expression | Uses parse-duration package to resolve next date. Available units: - milliseconds (ms) - seconds (s, sec) - minutes (m, min) - hours (h, hr) - days (d) - weeks (w, wk) - months - years (y, yr) | "5m 20s" |
number of milliseconds | Number of milliseconds in which should the task run again. | 5 * 60 * 1000 |
CRON expression | Has to be always prefixed by "CRON " string. Uses cron-parser package to resolve next date. It is possible to pass cronExpressionParserOptions object option to mongodash init, see Options in cron-parser package documentation for valid options. The endDate option is not supported yet. | "CRON */5 * * * *" |
Function (sync or async) | Function with any timing logic, which will be evaluated after each task run to schedule the next run. It should return instance of Date, interval expression, CRON expression or number of milliseconds. | () => new Date() () => "5m 20s" () => "CRON */5 * * * *" () => 5 * 60 * 1000 |
See a step by step task registration:
scheduleCronTaskImmediately(taskId) => Promise
scheduleCronTaskImmediately(taskId) => Promise
Schedule the task to run as soon as possible. Handy for cases when you need to hurry up a task based on another task or an API call.
const { scheduleCronTaskImmediately } = require('mongodash');
scheduleCronTaskImmediately('my-task-id');
import { scheduleCronTaskImmediately } from 'mongodash';
scheduleCronTaskImmediately('my-task-id');
runCronTask(taskId) => Promise
runCronTask(taskId) => Promise
Run a task and return a promise. The promise is resolved as soon as the task is done. The main purpose of the function is to easily test the tasks in automated tests.
const { runCronTask } = require('mongodash');
runCronTask('my-task-id');
Do not use the runCronTask method inside a cron task
It will not work. Use non-blocking scheduleCronTaskImmediately instead.
stopCronTasks() => void
stopCronTasks() => void
Stop triggering registered tasks. Useful for automated tests, where is usually not desired to run tasks in the background. Calling stopCronTasks function before the first cronTask registration will prevent running any task.
const { stopCronTasks } = require('mongodash');
stopCronTasks();
import { stopCronTasks } from 'mongodash';
stopCronTasks();
startCronTasks() => void
startCronTasks() => void
Start triggering registered tasks. Usually not needed to call, since the registered tasks run automatically after the registration unless stopCronTasks is called or the mongodash is initialized with runCronTasks: false.
const { startCronTasks } = require('mongodash');
startCronTasks(); // returns void
import { startCronTasks } from 'mongodash';
startCronTasks(); // returns void
Initialization options (optional)
const mongodash = require('mongodash');
mongodash.init({
// database connection
uri: 'mongodb://mongodb0.example.com:27017',
// true by default
runCronTasks: false,
// valid only if CRON expressions used
// see https://www.npmjs.com/package/cron-parser for valid options
cronExpressionParserOptions: {
tz: 'Europe/Athens',
},
});
import mongodash from 'mongodash';
mongodash.init({
// database connection
uri: 'mongodb://mongodb0.example.com:27017',
// true by default
runCronTasks: false,
// valid only if CRON expressions used
// see https://www.npmjs.com/package/cron-parser for valid options
cronExpressionParserOptions: {
tz: 'Europe/Athens',
},
});
Manual task run
Need to manually trigger a task outside the application? Mongodash Cron tasks allow you to speed up task processing by setting runImmediatelly
flag to true
. Just find and update the task document in cronTasks
collection. This is helpful in a deployment environment to speed up a process.
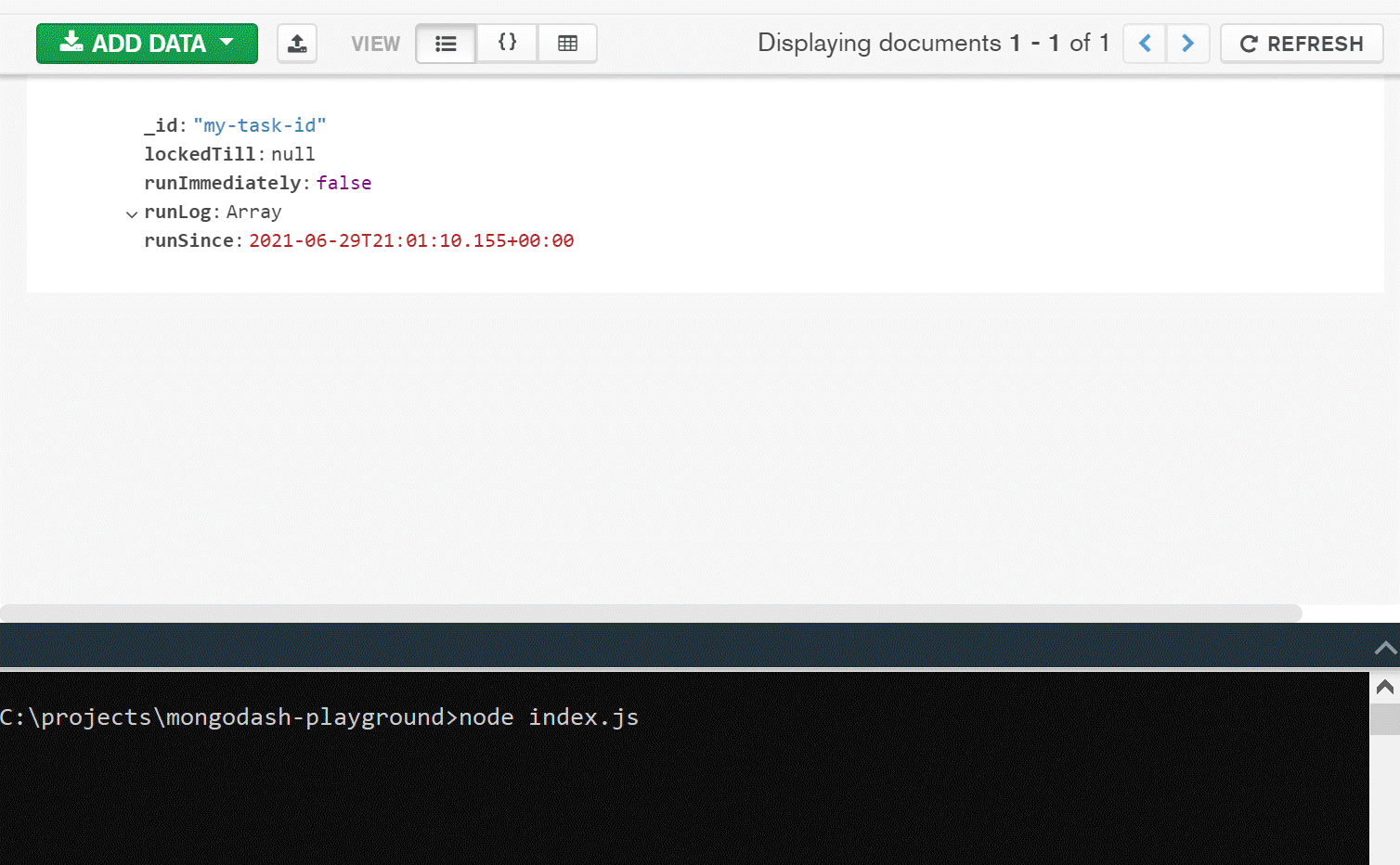
Long running tasks
missing documentation here
Automatic lock extension....
Updated over 3 years ago